챗봇에게 기억력 선사하기
=> 이전 질문에 이어지는 대답하기
=> 이전 대화의 내용 적용하기
컨텍스트 사용
랭체인에는 5가지 정도의 메모리가 있음
모델 자체(GPT 같은)에는 메모리 탑재 노노!
챗지피티에는 탑재
모델에게 이전 대화 전체를 보내는 형식으로 진행
모든 메모리는 동일한 api 형식 사용
save_context, load_memory_variables 등의 함수
🦜첫 번째 메모리 - ConversationBufferMemory
단순히 이전 대화 내용 전체를 저장
대화 내용이 길어질수록 비효율적임 ( 동일한 내용이 중복되어 쌓임)
예측을 할 때, 텍스트를 자동완성하고 싶을 때 유용
from langchain.memory import ConversationBufferMemory
memory = ConversationBufferMemory(return_messages=True) # 메모리 생성
# return_messages=True 옵션 안 넣으면 그냥 문자열 저장 => {'history': 'Human: Hi!\nAI: How are you?'}
# 챗모델이 사용할 수 있는 형태로 저장하려면 true
memory.save_context({"input": "Hi!"}, {
"output": "How are you?"}) # 사용자 입력,모델 출력 저장
memory.load_memory_variables({}) # 히스토리(대화 내용 ) 확인
🦜 두 번째 메모리 - ConversationBufferWindowMemory
대화의 특정 내용을 저장하는 메모리
최근 대화만 저장 (이전은 버림)
메모리 크기를 효율적으로 관리할 수 있음
예전 대화를 기억하기 힘들다는 단점
from langchain.memory import ConversationBufferWindowMemory
memory = ConversationBufferWindowMemory(
return_messages=True,
k=4, # 버퍼 윈도우의 사이즈 (저장할 메세지 크기)
)
🦜 세 번째 메모리 - ConversationBufferMemory
from langchain.chat_models import ChatOpenAI
llm = ChatOpenAI(temperature=0.1)
얘는 LLm을 사용
from langchain.memory import ConversationSummaryMemory
memory = ConversationSummaryMemory(llm=llm)
대화의 내용을 자체적으로 요약해서 저장
add_message("Hi I'm celina, I live in South Korea", "Wow that is so cool!")
add_message("South Kddorea is so pretty", "I wish I could go!!!")
=> {'history': 'Celina introduces herself as living in South Korea, which the AI finds cool. Celina mentions how pretty South Korea is, and the AI expresses a wish to visit.'}
이런 식으로 히스토리가 저장됨
처음에는 메모리를 많이 요할 수 있지만 대화가 길어질수록 효율적임
🦜 네 번째 메모리 - ConversationSummaryBufferMemory
메모리에 정해놓은 한계에 도달하면 이전 내용을 요약하여 정리
from langchain.memory import ConversationSummaryMemory
from langchain.chat_models import ChatOpenAI
llm = ChatOpenAI(temperature=0.1)
memory = ConversationSummaryMemory(llm=llm)
예전의 메세지까지 모두 요약돠어 정리됨
{'history': [HumanMessage(content="Hi I'm celina, I live in South Korea"), AIMessage(content='Wow that is so cool!')]}
이런 히스토리가, max_token_limit에 도달하면,
{'history': [SystemMessage(content='The human introduces themselves as Celina and mentions they live in South Korea. The AI responds with enthusiasm, saying that it is cool.'),
이렇게 바뀜
🦜 다섯 번째 메모리 - ConversationKGMemory
이것도 LLM 사용
대화 중의 엔티티의 knowledge graph 만들
요약 => 대화에서 entity 추출
from langchain.memory import ConversationKGMemory
from langchain.chat_models import ChatOpenAI
llm = ChatOpenAI(temperature=0.1)
memory = ConversationKGMemory(
llm=llm,
return_messages=True,
)
🦜 Memory on LLMChain
메모리를 체인에 꽂기
off - the-shell : 일반적인 목적을 가진 chain
또는 커스텀 체인 만들기 가능
메모리의 내용을 모델에게 전달
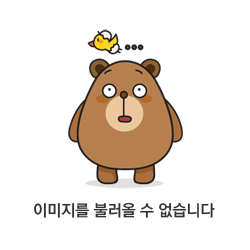
프롬프트에 보면 대화 내역이 저장되어 있
🦜Chat Based Memory
from langchain.prompts import ChatPromptTemplate, MessagesPlaceholder
memory = ConversationSummaryBufferMemory(
llm=llm,
max_token_limit=120,
memory_key="chat_history",
return_messages=True,
)
prompt = ChatPromptTemplate.from_messages(
[
("system", "You are a helpful AI talking to a human"),
MessagesPlaceholder(variable_name="chat_history"), # AI의 대답 부분을 이렇게 처리하기!
("human", "{question}"),
]
)
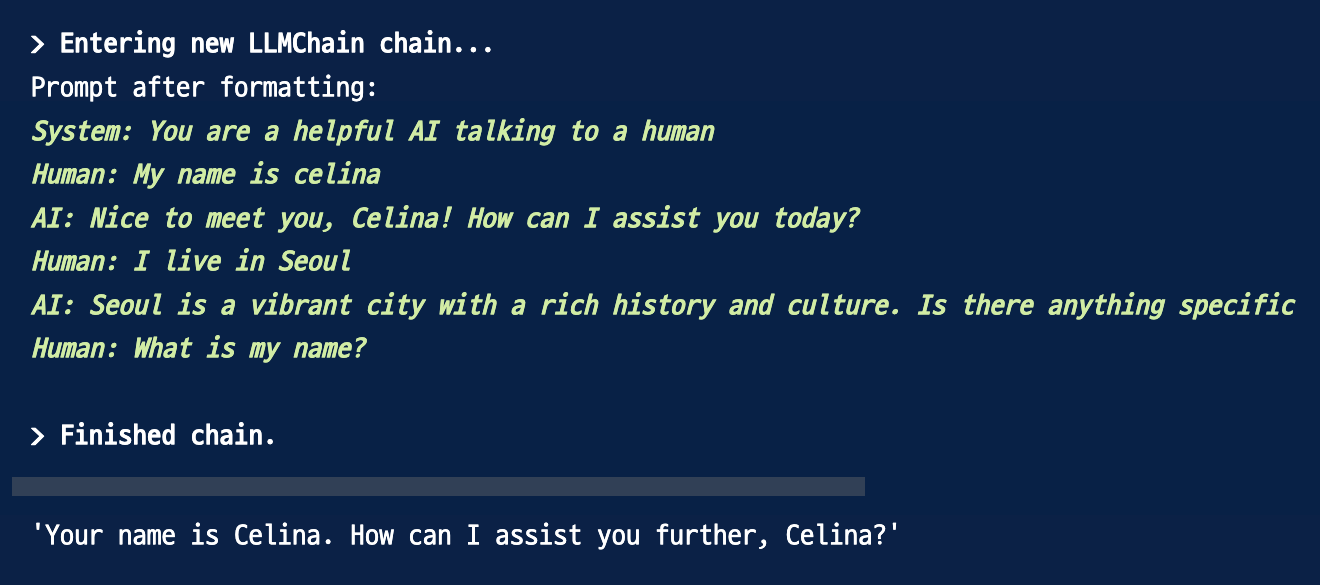
🦜 Based Memory
from langchain.memory import ConversationSummaryBufferMemory
from langchain.chat_models import ChatOpenAI
from langchain.schema.runnable import RunnablePassthrough
from langchain.prompts import ChatPromptTemplate, MessagesPlaceholder
llm = ChatOpenAI(temperature=0.1)
memory = ConversationSummaryBufferMemory(
llm=llm,
max_token_limit=120,
return_messages=True,
)
prompt = ChatPromptTemplate.from_messages(
[
("system", "You are a helpful AI talking to a human"),
MessagesPlaceholder(variable_name="history"),
("human", "{question}"),
]
)
def load_memory(_): # 메모리 변수들을 가져오는 함수
return memory.load_memory_variables({})["history"] # 메모리를 로드
chain = RunnablePassthrough.assign(
history=load_memory) | prompt | llm # 체인 만들기
def invoke_chain(question):
result = chain.invoke({"question": question}) # invoke
memory.save_context( # 사람과 AI의 메세지를 메모리에 저장
{"input": question},
{"output": result.content},
)
print(result)
'🤖 AI > LangChain - GPT 강의' 카테고리의 다른 글
🦜 풀스택 GPT - Quiz GPT ing (0) | 2024.05.28 |
---|---|
🦜 풀스택 GPT - RAG (0) | 2024.03.03 |
🦜 풀스택 GPT - MODEL IO (0) | 2024.02.20 |
🦜 풀스택 GPT - LangChain (2) | 2024.02.17 |
🦜 풀스택 GPT - 시작하기~ (0) | 2024.01.07 |